python實(shí)現(xiàn)過(guò)濾敏感詞
關(guān)于敏感詞過(guò)濾可以看成是一種文本反垃圾算法,例如 題目:敏感詞文本文件 filtered_words.txt,當(dāng)用戶輸入敏感詞語(yǔ),則用 星號(hào) * 替換,例如當(dāng)用戶輸入「北京是個(gè)好城市」,則變成「**是個(gè)好城市」 代碼:
#coding=utf-8def filterwords(x): with open(x,’r’) as f:text=f.read() print text.split(’n’) userinput=raw_input(’myinput:’) for i in text.split(’n’):if i in userinput: replace_str=’*’*len(i.decode(’utf-8’)) word=userinput.replace(i,replace_str) return wordprint filterwords(’filtered_words.txt’)
再例如反黃系列:
開(kāi)發(fā)敏感詞語(yǔ)過(guò)濾程序,提示用戶輸入評(píng)論內(nèi)容,如果用戶輸入的內(nèi)容中包含特殊的字符:敏感詞列表 li = ['蒼老師','東京熱',”武藤蘭”,”波多野結(jié)衣”]則將用戶輸入的內(nèi)容中的敏感詞匯替換成***,并添加到一個(gè)列表中;如果用戶輸入的內(nèi)容沒(méi)有敏感詞匯,則直接添加到上述的列表中。content = input(’請(qǐng)輸入你的內(nèi)容:’)li = ['蒼老師','東京熱','武藤蘭','波多野結(jié)衣']i = 0while i < 4: for li[i] in content:li1 = content.replace(’蒼老師’,’***’)li2 = li1.replace(’東京熱’,’***’)li3 = li2.replace(’武藤蘭’,’***’)li4 = li3.replace(’波多野結(jié)衣’,’***’) else:pass i += 1
一道bat面試題:快速替換10億條標(biāo)題中的5萬(wàn)個(gè)敏感詞,有哪些解決思路? 有十億個(gè)標(biāo)題,存在一個(gè)文件中,一行一個(gè)標(biāo)題。有5萬(wàn)個(gè)敏感詞,存在另一個(gè)文件。寫(xiě)一個(gè)程序過(guò)濾掉所有標(biāo)題中的所有敏感詞,保存到另一個(gè)文件中。
1、DFA過(guò)濾敏感詞算法
在實(shí)現(xiàn)文字過(guò)濾的算法中,DFA是比較好的實(shí)現(xiàn)算法。DFA即Deterministic Finite Automaton,也就是確定有窮自動(dòng)機(jī)。 算法核心是建立了以敏感詞為基礎(chǔ)的許多敏感詞樹(shù)。 python 實(shí)現(xiàn)DFA算法:
# -*- coding:utf-8 -*-import timetime1=time.time()# DFA算法class DFAFilter(): def __init__(self):self.keyword_chains = {}self.delimit = ’x00’ def add(self, keyword):keyword = keyword.lower()chars = keyword.strip()if not chars: returnlevel = self.keyword_chainsfor i in range(len(chars)): if chars[i] in level:level = level[chars[i]] else:if not isinstance(level, dict): breakfor j in range(i, len(chars)): level[chars[j]] = {} last_level, last_char = level, chars[j] level = level[chars[j]]last_level[last_char] = {self.delimit: 0}breakif i == len(chars) - 1: level[self.delimit] = 0 def parse(self, path):with open(path,encoding=’utf-8’) as f: for keyword in f:self.add(str(keyword).strip()) def filter(self, message, repl='*'):message = message.lower()ret = []start = 0while start < len(message): level = self.keyword_chains step_ins = 0 for char in message[start:]:if char in level: step_ins += 1 if self.delimit not in level[char]:level = level[char] else:ret.append(repl * step_ins)start += step_ins - 1breakelse: ret.append(message[start]) break else:ret.append(message[start]) start += 1return ’’.join(ret)if __name__ == '__main__': gfw = DFAFilter() path='F:/文本反垃圾算法/sensitive_words.txt' gfw.parse(path) text='新疆騷亂蘋(píng)果新品發(fā)布會(huì)?八' result = gfw.filter(text) print(text) print(result) time2 = time.time() print(’總共耗時(shí):’ + str(time2 - time1) + ’s’)
運(yùn)行效果:
新疆騷亂蘋(píng)果新品發(fā)布會(huì)?八****蘋(píng)果新品發(fā)布會(huì)**總共耗時(shí):0.0010344982147216797s
2、AC自動(dòng)機(jī)過(guò)濾敏感詞算法
AC自動(dòng)機(jī):一個(gè)常見(jiàn)的例子就是給出n個(gè)單詞,再給出一段包含m個(gè)字符的文章,讓你找出有多少個(gè)單詞在文章里出現(xiàn)過(guò)。 簡(jiǎn)單地講,AC自動(dòng)機(jī)就是字典樹(shù)+kmp算法+失配指針
# -*- coding:utf-8 -*-import timetime1=time.time()# AC自動(dòng)機(jī)算法class node(object): def __init__(self):self.next = {}self.fail = Noneself.isWord = Falseself.word = ''class ac_automation(object): def __init__(self):self.root = node() # 添加敏感詞函數(shù) def addword(self, word):temp_root = self.rootfor char in word: if char not in temp_root.next:temp_root.next[char] = node() temp_root = temp_root.next[char]temp_root.isWord = Truetemp_root.word = word # 失敗指針函數(shù) def make_fail(self):temp_que = []temp_que.append(self.root)while len(temp_que) != 0: temp = temp_que.pop(0) p = None for key,value in temp.next.item():if temp == self.root: temp.next[key].fail = self.rootelse: p = temp.fail while p is not None:if key in p.next: temp.next[key].fail = p.fail breakp = p.fail if p is None:temp.next[key].fail = self.roottemp_que.append(temp.next[key]) # 查找敏感詞函數(shù) def search(self, content):p = self.rootresult = []currentposition = 0while currentposition < len(content): word = content[currentposition] while word in p.next == False and p != self.root:p = p.fail if word in p.next:p = p.next[word] else:p = self.root if p.isWord:result.append(p.word)p = self.root currentposition += 1return result # 加載敏感詞庫(kù)函數(shù) def parse(self, path):with open(path,encoding=’utf-8’) as f: for keyword in f:self.addword(str(keyword).strip()) # 敏感詞替換函數(shù) def words_replace(self, text):''':param ah: AC自動(dòng)機(jī):param text: 文本:return: 過(guò)濾敏感詞之后的文本'''result = list(set(self.search(text)))for x in result: m = text.replace(x, ’*’ * len(x)) text = mreturn textif __name__ == ’__main__’: ah = ac_automation() path=’F:/文本反垃圾算法/sensitive_words.txt’ ah.parse(path) text1='新疆騷亂蘋(píng)果新品發(fā)布會(huì)?八' text2=ah.words_replace(text1) print(text1) print(text2) time2 = time.time() print(’總共耗時(shí):’ + str(time2 - time1) + ’s’)
運(yùn)行結(jié)果:
新疆騷亂蘋(píng)果新品發(fā)布會(huì)?八****蘋(píng)果新品發(fā)布會(huì)**總共耗時(shí):0.0010304450988769531s
以上就是python實(shí)現(xiàn)過(guò)濾敏感詞的詳細(xì)內(nèi)容,更多關(guān)于python 過(guò)濾敏感詞的資料請(qǐng)關(guān)注好吧啦網(wǎng)其它相關(guān)文章!
相關(guān)文章:
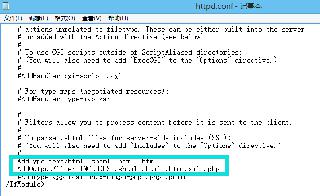